File encryption in Laravel
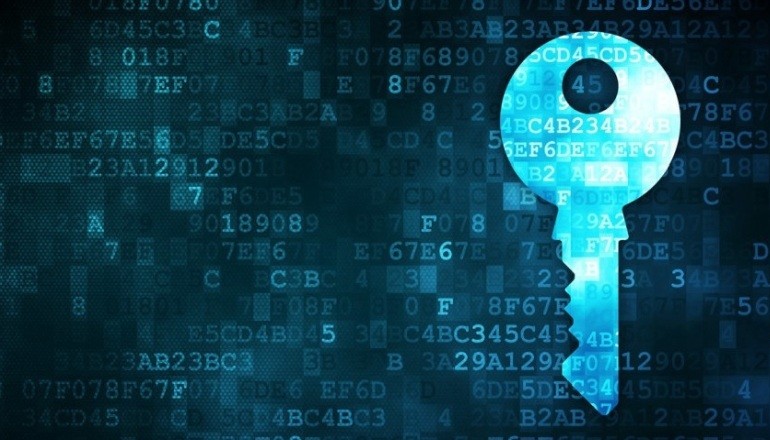
When it is necessary to encrypt a string in Laravel, it is sufficient to use encrypt () helper and if you had the task of saving sensitive information to the database, then you have certainly used encrypt () helper. But what about encrypting readable files in Laravel?
Sometimes this is also necessary, depending on the tasks of the application, but if you are saving medical findings or similar files, then due to patient privacy and data security, accompanying documentation in the form of JPG images and PDF files, which are saved to disk / storage , encrypt and protect against unauthorized access. Unauthorized access does not necessarily mean that access to a web application or web server is compromised by unwanted third parties, it can simply be a situation that a certain rank of users cannot have access to these files, such as. server administrator and you want to ensure that access to these files is controlled through a web application.
Believe it or not, the solution in Laravel lies in using the already mentioned encrypt ()
helper function. Yes, the same ones you use to encrypt sensitive data / columns in your database.
public function store()
{
// Get file content in memory
$fileContent = Storage::get('files/report.pdf');
// Encrypt the File Content
$encryptedContent = encrypt($fileContent);
// Store the encrypted Content on Storage
Storage::put('files/report.pdf', $encryptedContent);
}
Of course, in order for the file to be read / opened, it is necessary to decrypt it and offer it for download.
public function download()
{
$filePath = 'files/report.pdf';
$decryptedContent = decrypt(Storage::get($filePath));
return \Response::make($decryptedContent, 200, [
'Cache-Control' => 'must-revalidate, post-check=0, pre-check=0',
'Content-Type' => Storage::mimeType($filePath),
'Content-Length' => Storage::size($filePath),
'Content-Disposition' => 'attachment; filename="' . basename($filePath) . '"',
'Pragma' => 'public',
]);
}
Laravel uses OpenSSL to enable AES-256 and AES-128 encryption, and it should be noted that encrypted files will take up more disk space than the original files.